Are you eager to dive into the world of data analysis and explore the possibilities that R programming has to offer? Then understanding the syntax of R is your essential first step. The syntax of a programming language determines how code is written and executed, making it the backbone of any coding endeavor. So, what exactly is the syntax of R programming?
In this comprehensive guide, we will take you on a journey through the syntax of R programming, unraveling its intricacies and empowering you to write efficient code. Whether you are a beginner or an experienced coder, this article will equip you with the knowledge and skills needed to leverage the power of R for data analysis and beyond.
Table of Contents
- Introduction to R Programming
- An Overview of R Programming
- R Basics: Variables, Data Types, and Operators
- Key Concepts Covered in this Section:
- R Syntax Basics
- Variables and Data Types in R
- Control Structures in R
- Functions in R Programming
- Working with R Data Structures
- Input and Output in R
- Reading Data
- Writing Data
- Working with Files and Databases
- File Operations and Database Connectivity in R
- Error Handling and Debugging in R
- Working with Packages and Libraries in R
- Data Visualization in R
- Advanced Topics in R Programming
- Conclusion
- FAQ
- What is R programming?
- What are the basics of R programming?
- How can I declare and assign values to variables in R?
- What are the control structures in R?
- How do I define and call functions in R?
- What are the different data structures in R?
- How can I read data from external sources in R?
- How do I handle errors and debug code in R?
- How do I install and load packages in R?
- What are some widely used packages for data visualization in R?
- What are some advanced topics in R programming?
Key Takeaways:
- Understanding the syntax of R programming is crucial for writing efficient code.
- The syntax of R determines how code is written and executed.
- Mastering R syntax opens doors to advanced data analysis techniques and statistical modeling.
- R syntax includes elements such as variables, data types, control structures, and functions.
- Proper coding conventions enhance code readability and maintainability.
Introduction to R Programming
In this section, we delve deeper into the fundamentals of R programming, providing an essential introduction to this powerful programming language. R is widely used in data analysis, statistics, and research, making it a valuable tool for professionals in various fields.
R is a versatile and extensible language that is not only easy to learn but also highly efficient in handling large data sets. With a wide range of built-in functions and packages, R offers endless possibilities for data manipulation, visualization, and modeling.
R has gained popularity among data scientists, statisticians, and researchers due to its open-source nature and extensive community support. It provides a user-friendly environment for analyzing and visualizing data, making it an ideal choice for both beginners and experienced programmers.
An Overview of R Programming
R is a powerful programming language designed specifically for statistical computing and graphics. It provides a comprehensive set of tools for data analysis, making it a preferred choice for professionals in fields such as finance, healthcare, marketing, and social sciences.
One of the key advantages of R is its ability to handle a wide range of data types and formats. From numeric and character data to complex data structures like matrices and lists, R can effectively handle diverse data sources and perform advanced data manipulations.
R also offers a vast collection of packages and libraries that extend its functionality. These packages cover various domains, including machine learning, data visualization, and statistical analysis. By leveraging these packages, programmers can accelerate their development process and access advanced tools and techniques.
R Basics: Variables, Data Types, and Operators
Before diving into R programming, it is crucial to familiarize yourself with the basic concepts. Understanding variables, data types, and operators in R is essential for writing effective and efficient code.
In R, variables are used to store and manipulate data. They can hold different types of data, including numbers, strings (text), logical values (TRUE or FALSE), and more. By assigning values to variables, you can perform calculations, create conditional statements, and store results for future use.
R supports a wide range of operators that allow performing various operations on data. From arithmetic operators for basic calculations to logical operators for comparing values, R provides a comprehensive set of tools for data manipulation and analysis.
Key Concepts Covered in this Section:
- The purpose, features, and advantages of R programming
- Introduction to variables, data types, and operators in R
- Overview of R programming for statistical computing and graphics
- Understanding the extensive collection of packages and libraries in R
R Syntax Basics
In this section, we dive into the fundamental syntax of R programming. Understanding the R syntax is essential for writing efficient and readable code. From writing R statements to following coding conventions, we cover all the bases to help you become proficient in R programming.
R Statements
In R programming, statements are individual instructions that perform specific actions. Each statement ends with a newline character or a semicolon (;). You can write multiple statements on a single line, separated by semicolons, but it’s best practice to write each statement on a new line for better code readability.
“Always remember, a single misplaced semicolon can cause syntax errors!”
Let’s take a look at an example of an R statement:
# Assigning a value to a variable
x
In the example above, the statement assigns the value 10 to the variable x using the assignment operator (<-
). The “#” symbol indicates a comment, which is ignored by R and is used to add explanatory notes to your code.
Coding Conventions
Coding conventions are guidelines that help developers write consistent, readable, and maintainable code. Although R is a flexible language, following coding conventions can greatly enhance the readability of your code and make it easier to collaborate with others.
- Variable and function names should be descriptive and meaningful.
- Use lowercase letters and separate words in variable names with underscores (_). For example,
total_sales
. - Indent your code properly to improve readability.
- Use spaces around operators and before opening parentheses for better clarity.
- Comment your code to provide insights and explanations.
R Coding Conventions
Convention | Example |
---|---|
Variable Naming | customer_name |
Function Naming | calculate_sales |
Indentation | – |
Operator Spacing | x |
Commenting | # Calculate total sales |
By adhering to these coding conventions, you can write code that is not only functional but also easy to understand and maintain.
Variables and Data Types in R
In the world of R programming, variables and data types are fundamental concepts that form the building blocks of any code. Understanding how to declare and assign values to variables, as well as working with different data types, is essential for effective data analysis and manipulation.
Variable Declaration and Assignment:
In R, variables are created by assigning a value to a name, using the assignment operator (<-
or =
). For example:
x <- 10
name <- "John Doe"
Data Types in R:
R supports several data types, including numeric, character, and logical.
- Numeric: Numeric data types in R represent numbers, both integers and decimals. They are commonly used for quantitative measurements, such as ages, heights, and stock prices.
- Character: Character data types store text and are represented by enclosing the text in single (‘ ‘) or double quotes (” “). They are used to store names, addresses, descriptions, and other textual information.
- Logical: Logical data types in R represent binary values, either
TRUE
orFALSE
. They are used for logical operations and control flow, such as conditional statements and loops.
Here’s an example demonstrating the usage of different data types in R:
Variable | Data Type | Value |
---|---|---|
x | Numeric | 10 |
name | Character | “John Doe” |
is_true | Logical | TRUE |
By understanding variables and data types in R, you gain the foundation needed to work with data effectively, perform calculations, and write code that yields accurate and meaningful results.
Control Structures in R
Control structures are fundamental elements in programming that allow the flow of execution to be altered based on certain conditions or iterations. In R, there are several control structures that facilitate decision-making and looping, including conditional statements, loops, and branching.
Conditional Statements
Conditional statements are used to evaluate specific conditions and execute different blocks of code based on the outcome. In R, the most commonly used conditional statement is the if-else statement. It allows the program to execute one set of statements if the condition is true and another set of statements if the condition is false. Here’s an example:
if(condition){ # code block executed if condition is true } else{ # code block executed if condition is false }
R also supports nested if-else statements, where one if-else statement is inside another. This allows for more complex decision-making in the code.
Loops
Loops are used to repeat a specific block of code multiple times. They are beneficial when you need to perform the same operation iteratively. R provides three types of loops: for loop, while loop, and repeat loop.
The for loop is commonly used when you know the exact number of iterations. It iterates over a sequence or a collection of elements. Here’s an example:
for (variable in sequence){ # code block executed for each element in the sequence }
The while loop is useful when you want to keep iterating until a specific condition becomes false. It repeatedly executes a block of code as long as the condition remains true. Here’s an example:
while (condition){ # code block executed as long as the condition is true }
The repeat loop is used when you need to execute a block of code indefinitely until a specific condition is met. It uses the break statement to exit the loop. Here’s an example:
repeat { # code block executed until break statement if (condition){ break } }
Branching
Branching is the process of selecting a specific path based on certain conditions or values. In R, the switch statement allows you to select one of several code blocks to execute based on the value of an expression. It provides an efficient alternative to using multiple if-else statements. Here’s an example:
switch(expression, value1 = { # code block executed when expression is value1 }, value2 = { # code block executed when expression is value2 }, value3 = { # code block executed when expression is value3 }, default = { # code block executed when expression doesn't match any value } )
By understanding and utilizing control structures, R programmers gain the ability to control program execution flow, make decisions based on conditions, and repeat code blocks as needed. The effective use of control structures can enhance the efficiency and logic of R programs.
Control Structure | Description | Example |
---|---|---|
if-else | Executes different blocks of code based on a condition | if(condition){ # code block executed if condition is true } else{ # code block executed if condition is false } |
for | Repeats a block of code for each element in a sequence | for (variable in sequence){ # code block executed for each element in the sequence } |
while | Repeats a block of code as long as a condition is true | while (condition){ # code block executed as long as the condition is true } |
repeat | Repeats a block of code indefinitely until a break statement is encountered | repeat { # code block executed until break statement if (condition){ break } } |
switch | Selects one of several code blocks to execute based on the value of an expression | switch(expression, value1 = { # code block executed when expression is value1 }, value2 = { # code block executed when expression is value2 }, value3 = { # code block executed when expression is value3 }, default = { # code block executed when expression doesn't match any value } ) |
Functions in R Programming
Functions play a key role in R programming. They allow you to encapsulate tasks and create reusable code, making your programs more modular and efficient. In this section, we will explore the world of functions in R, learning how to define and call functions, pass arguments, and handle return values.
Function Definition
A function in R is defined using the function
keyword followed by the function name, parentheses for arguments, and curly braces for the function body. Here is the general syntax:
function_name
# Function body
# Code goes here
# Return value (optional)
}
Let’s take a closer look at each component:
- function_name: Choose a descriptive name for your function that reflects its purpose.
- arg1, arg2, …: These are the function’s arguments, which are inputs passed to the function. You can have zero or more arguments.
- Function body: This is where you write the code that defines the behavior of your function. It can include any valid R code.
- Return value: In R, the return value of a function is the value computed by the function. It is optional, as some functions may not need to return a value.
Function Call
To use a function in R, you need to call it by its name and provide any required arguments. Here is the general syntax for function calls:
result
Let’s break down the syntax:
- result: Assign the result of the function call to a variable. This allows you to store and manipulate the returned value.
- function_name: Specify the name of the function you want to call.
- arg1, arg2, …: Provide the required arguments for the function. These values will be used as inputs in the function’s code.
Here’s an example of a function definition and call:
# Function definition
multiply
result
return(result)
}# Function call
product
print(product) # Output: 15
Arguments and Return Values
In R, functions can have different types of arguments, such as required arguments, default arguments, and variable-length arguments. You can also specify the return value of a function using the return
keyword. Here are some examples:
# Function with required arguments
calculate_area
area
return(area)
}# Function with default arguments
greet_user
message
return(message)
}# Function with variable-length arguments
sum_numbers
numbers
total
return(total)
}
By understanding how to define functions, call them with appropriate arguments, and handle their return values, you can leverage the power of functions to streamline your R programs and enhance code reusability.
Working with R Data Structures
In the world of data analysis and manipulation, R offers a powerful set of data structures to handle and organize data effectively. Understanding these data structures and knowing when to use them can significantly enhance your productivity as a data scientist. In this section, we will explore the key data structures in R, including vectors, matrices, lists, and data frames, highlighting their usage and advantages in different scenarios.
R Vectors
A vector is the simplest and most common data structure in R. It is essentially a one-dimensional array that can store elements of the same data type, such as numbers, characters, or logical values. Vectors can be created using the c()
function, where elements are separated by commas. They provide a convenient way to store and manipulate collections of values.
R Matrices
A matrix is a two-dimensional data structure in R, consisting of rows and columns. It is created by combining vectors of equal length using the matrix()
function. Matrices are particularly useful for performing mathematical operations and matrix algebra. They are commonly employed in linear algebra, statistical modeling, and data analysis.
R Lists
A list is a versatile and flexible data structure in R that can contain elements of different types, including vectors, matrices, and even other lists. Lists are created using the list()
function and provide a convenient way to organize and manage complex data structures. They are commonly used for hierarchical or nested data, such as representing a dataset with multiple variables and attributes.
R Data Frames
A data frame is a tabular data structure in R, similar to a spreadsheet or a database table. It is a two-dimensional object that organizes data into rows and columns, where each column can have a different data type. Data frames are created using functions like read.csv()
or by converting matrices or lists into data frames. They are widely used for data manipulation, analysis, and modeling, providing a convenient way to work with structured data.
“Understanding and effectively working with R data structures is fundamental to performing data analysis efficiently and accurately. By harnessing the power of vectors, matrices, lists, and data frames, you can organize and manipulate data with ease, unlocking the full potential of the R programming language.”
Input and Output in R
Data input and output are essential processes in data analysis using R. This section focuses on how to read data from external sources, such as CSV files, as well as how to export data from R. It also covers basic file operations and working with databases.
Reading Data
When working with R, it is common to need to read data from external sources. The following methods can be used to import data into R:
- Using the read.table() function, which allows you to read data from a variety of formats, including CSV files, text files, and Excel spreadsheets.
- Using the read.csv() function specifically designed to read data from comma-separated values (CSV) files.
- Using the read_excel() function from the readxl package to read data from Excel files.
Here’s an example of how to read a CSV file using the read.csv() function:
data
Writing Data
After analyzing data in R, it may be necessary to export the results or save data for future use. The following methods can be used to export data from R:
- Using the write.table() function to save data to a text file.
- Using the write.csv() function to save data to a CSV file.
- Using the write.xlsx() function from the openxlsx package to save data to an Excel file.
Here’s an example of how to save data to a CSV file using the write.csv() function:
write.csv(data, "output.csv", row.names = FALSE)
Working with Files and Databases
Besides reading and writing data from files, R also allows working with files and databases directly. This can be useful when dealing with large datasets or when data is stored in a database.
Some common operations for working with files and databases in R include:
- Opening and closing files using the file() and close() functions.
- Checking if a file exists using the file.exists() function.
- Creating directories using the dir.create() function.
- Working with different file formats, such as PDF, XML, and JSON.
- Connecting to databases using database-specific packages like DBI and RSQLite.
When working with databases, R provides various packages tailored to specific database systems, such as RMySQL for MySQL databases and RPostgreSQL for PostgreSQL databases.
File Operations and Database Connectivity in R
Operation | Description | Function |
---|---|---|
Opening a file | Opens a file for reading or writing | file() |
Closing a file | Closes an open file | close() |
Checking file existence | Checks if a file exists | file.exists() |
Creating directories | Creates a new directory | dir.create() |
Working with different file formats | Manipulating files in various formats (PDF, XML, JSON, etc.) | Package-specific functions |
Connecting to databases | Establishes a connection to a database | Package-specific functions (e.g., RMySQL, RPostgreSQL) |
Error Handling and Debugging in R
While programming in R, encountering errors and debugging code is a common occurrence. Therefore, understanding effective error handling techniques and troubleshooting strategies is crucial for developers to identify and resolve issues efficiently.
When errors occur in R, the interpreter displays error messages that provide valuable insights into the problem at hand. These error messages contain information such as the nature of the error, the line number where it occurred, and relevant contextual details. By carefully analyzing these error messages, developers can pinpoint the root cause of the issue and proceed with the necessary debugging steps.
Error handling in R involves implementing appropriate measures to gracefully handle errors, preventing program termination or unexpected behavior. This can include using constructs like try-catch blocks to catch and handle specific types of errors, ensuring program stability even in the presence of unforeseen issues.
To effectively troubleshoot and debug R code, developers can employ various techniques such as:
- Using print statements or message functions strategically to gain insight into the program’s execution and values of variables at different points.
- Using the browser() function to pause the program’s execution at specific breakpoints, allowing developers to interactively examine the program’s state.
- Using debugging tools like breakpoints and step-by-step execution to systematically analyze the code’s flow and identify potential issues.
- Inspecting intermediate results, variable values, and intermediate calculations to identify any discrepancies or unexpected behavior.
By incorporating these error handling and debugging techniques into their workflow, developers can streamline the development and troubleshooting process, ensuring more robust and reliable R programs.
Working with Packages and Libraries in R
R, with its extensive collection of packages and libraries, provides users with a vast array of tools and functionalities to enhance their data analysis capabilities. In this section, we will explore how to effectively install and load packages in R, as well as provide an overview of some essential libraries that are frequently used for data analysis.
Installing Packages
Before utilizing the power of R packages, it is necessary to install them. Thankfully, installing packages in R is a straightforward process. Users can leverage the install.packages()
function to download packages from CRAN (Comprehensive R Archive Network), the official repository for R packages. Additionally, users can also install packages from other sources, such as GitHub, using the devtools
package.
“Installing packages in R is a breeze. With a simple function call, users can access a vast library of tools and functionalities to supercharge their data analysis projects.”
Loading Libraries
Once packages are installed, they need to be loaded into the R environment so that their functions and features can be accessed. The library()
function is used to load packages in R. By loading a package, its functions, datasets, and other resources become available for immediate use.
It is important to note that some packages have dependencies on other packages. When a package is loaded, R will automatically load its dependencies, ensuring that all required resources are available. However, if a package conflicts with another package already loaded, a warning message will be displayed, and users may need to manage the conflicts manually.
“Loading packages in R is the gateway to unlocking their functionalities. Once loaded, users can tap into a vast ecosystem of libraries to streamline their data analysis workflows.”
Data Visualization in R
Visualizing data plays a critical role in gaining insights and effectively communicating results. In R, there are several powerful tools and libraries available for creating visually appealing and informative plots and charts. One of the most popular and widely used packages for data visualization in R is ggplot2.
ggplot2 is an advanced plotting system that allows users to create a wide variety of plots with ease. It follows the principle of “grammar of graphics,” enabling users to construct plots by combining different layers, aesthetics, and statistical transformations.
With ggplot2, you can create various types of plots and charts, including scatter plots, bar charts, line graphs, histograms, boxplots, and more. This package provides a flexible and intuitive syntax that allows for easy customization and modification of visual elements.
Let’s take a look at a few examples of data visualization using ggplot2:
Example 1: Scatter Plot
A scatter plot is a useful visualization for displaying the relationship between two continuous variables. It can help identify patterns, correlations, and outliers within the data.
“The scatter plot below represents the relationship between the average temperature (in degrees Celsius) and the sales volume (in units) for a retail store over a period of one year.”
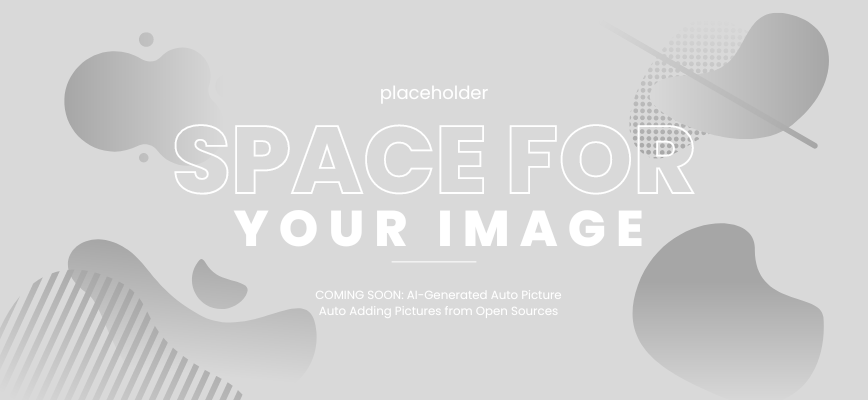
Example 2: Bar Chart
A bar chart is a common visualization for comparing categorical data. It is particularly useful for showing the distribution of a variable across different categories or groups.
“The bar chart below illustrates the sales performance (in dollars) of different products in a retail store during the last quarter.”
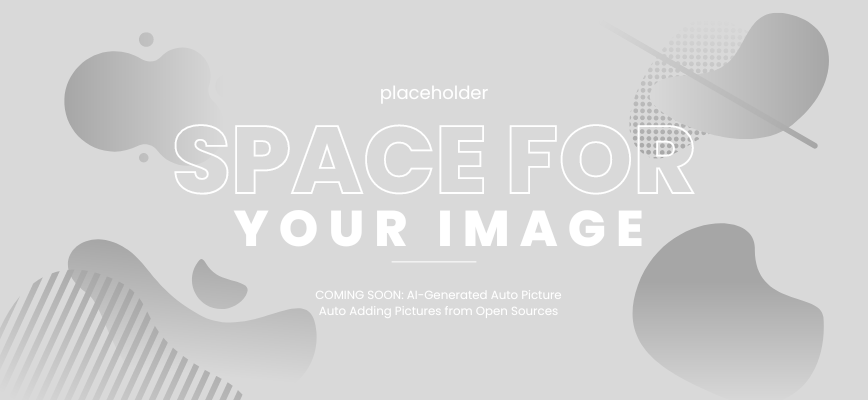
Example 3: Line Graph
A line graph is ideal for visualizing trends and changes over time. It can be used to represent continuous data and highlight patterns, fluctuations, or growth.
“The line graph below depicts the stock market prices (in dollars) of a particular company over a five-year period.”
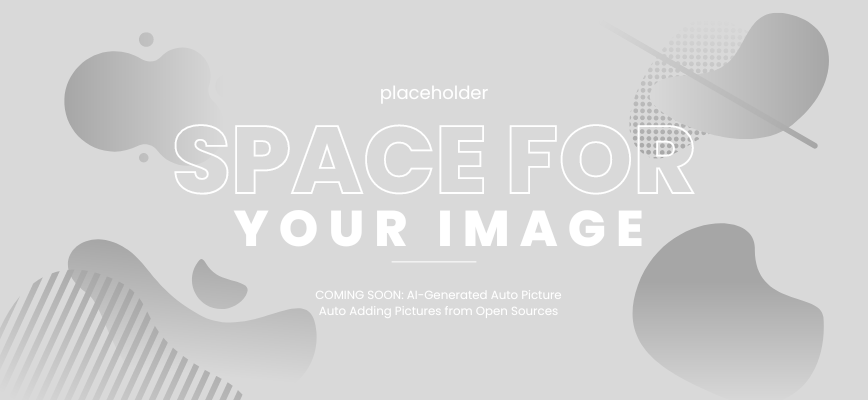
These examples demonstrate just a fraction of what can be achieved with ggplot2 in terms of data visualization in R. By utilizing the package’s powerful features and customization options, you can create visually compelling charts and plots that effectively convey your data’s story.
Next, we will explore how to use ggplot2 to create these and other types of plots, as well as learn more about styling and customizing visualizations.
Chart Type | Use Case | Key Features |
---|---|---|
Scatter Plot | Visualizing relationships between continuous variables | Points representing data, trend lines, labels |
Bar Chart | Comparing categorical data | Rectangular bars, axis labels, groupings |
Line Graph | Visualizing trends over time | Connected lines, markers, axes |
Advanced Topics in R Programming
In this section, we delve into advanced topics in R programming, exploring statistical analysis, machine learning, and other specialized areas. These topics are crucial for taking your skills in R programming to the next level and unlocking its full potential in data analysis and decision-making.
Statistical Analysis
R is widely used for statistical analysis due to its robust capabilities and extensive libraries. With R, you can perform various statistical tests and analyses, including descriptive statistics, hypothesis testing, regression analysis, time series analysis, and more. R provides a comprehensive suite of functions and packages specifically designed for statistical modeling and inference, making it a popular choice among statisticians and data analysts.
Machine Learning
Machine learning is a rapidly evolving field, and R offers powerful tools and frameworks for developing machine learning models. With R, you can explore and implement various machine learning algorithms, including classification, regression, clustering, and dimensionality reduction. R’s extensive collection of packages, such as caret, randomForest, and xgboost, empowers you to build predictive models, perform feature selection, conduct model evaluation, and handle complex data analysis tasks.
Other Specialized Areas
In addition to statistical analysis and machine learning, R supports various other specialized areas within data science. These include but are not limited to:
- Time Series Analysis: R provides a range of techniques for analyzing and forecasting time series data, enabling you to uncover patterns and make predictions for time-dependent data.
- Text Mining and Natural Language Processing: R offers libraries such as tm and tidytext that facilitate text mining and natural language processing tasks, allowing you to extract insights from unstructured text data.
- Network Analysis: R’s igraph library enables the analysis of complex networks and graphs, making it useful for social network analysis, network visualization, and network-based modeling.
- Web Scraping: R has packages like rvest and httr that facilitate web scraping, allowing you to collect data from websites and APIs for further analysis.
- Data Visualization: While covered in another section, R’s advanced graphics capabilities, exemplified by the ggplot2 package, enable the creation of visually compelling and informative data visualizations.
By exploring these advanced topics in R programming, you can expand your data analysis repertoire and tackle more complex problems. Remember, continued learning and practice are essential in mastering these topics, and there are abundant resources available to further explore and hone your skills in advanced R programming.
Advantages of R in Advanced Topics | Challenges in Advanced R |
---|---|
R provides an extensive collection of packages for advanced statistical analysis and machine learning tasks. | Advanced R programming often requires a solid understanding of statistical concepts and algorithms. |
R’s flexibility allows for seamless integration with other programming languages such as Python and C++, enhancing its capabilities in complex data analysis tasks. | Complex topics like machine learning may require substantial computational resources and expertise. |
R’s active and vibrant community ensures ongoing development and support for advanced techniques and packages. | Implementing advanced techniques in R may require careful data preprocessing and handling of large datasets. |
Conclusion
In conclusion, this comprehensive guide has provided a thorough overview of the syntax of R programming, ranging from the basics to advanced topics. By acquiring a deep understanding of the fundamental concepts, you will be able to enhance your data analysis skills and harness the full potential of R programming.
Throughout this guide, we explored the syntax and structure of R code, learned how to declare variables and work with different data types in R, and gained insights into control structures and functions. We also touched upon important topics like error handling, working with packages and libraries, data visualization, and advanced techniques such as statistical analysis and machine learning.
By mastering the syntax of R programming, you can unlock a world of possibilities for data analysis and manipulation. Whether you are a beginner starting your journey in R or an experienced programmer looking to expand your skillset, this guide has equipped you with the necessary knowledge to succeed.
Remember to practice and apply what you have learned in real-world scenarios. Additionally, continue exploring and leveraging resources like online communities, forums, and documentation to further sharpen your R programming skills. With dedication and perseverance, you can become proficient in R programming and excel in your data analysis endeavors.
FAQ
What is R programming?
R programming is a language primarily used for statistical analysis and data visualization. It provides a wide range of tools and libraries for data manipulation, modeling, and graphing.
What are the basics of R programming?
The basics of R programming include understanding variables, data types, and operators. It also involves writing and executing R statements and following coding conventions for clear and readable code.
How can I declare and assign values to variables in R?
To declare and assign values to variables in R, you can use the assignment operator ”
What are the control structures in R?
Control structures in R include if-else statements, for and while loops, and switch cases. They allow you to control the flow of execution based on certain conditions or iterate over a set of values.
How do I define and call functions in R?
In R, you can define functions using the “function” keyword, followed by the function name, arguments, and the body of the function. To call a function, you simply use its name and provide the necessary arguments.
What are the different data structures in R?
R offers various data structures, such as vectors, matrices, lists, and data frames. Vectors are used to store homogeneous data, matrices for two-dimensional data, lists for heterogeneous data, and data frames for tabular data.
How can I read data from external sources in R?
To read data from external sources, such as CSV files, you can use functions like “read.csv()” or “read.table()”. These functions allow you to import data into R for further analysis.
How do I handle errors and debug code in R?
Error handling in R involves using try-catch blocks to handle specific errors or using functions like “stop()” to generate custom error messages. For debugging, you can use tools like “debug()” or “traceback()” to identify and fix issues in your code.
How do I install and load packages in R?
To install packages in R, you can use the “install.packages()” function. Once installed, you can load packages using the “library()” function, which makes the functions and features of the package available for use in your R session.
What are some widely used packages for data visualization in R?
One popular package for data visualization in R is ggplot2. It provides a powerful and flexible framework for creating a wide range of plots and charts. Other popular packages include plotly, lattice, and ggvis.
What are some advanced topics in R programming?
Advanced topics in R programming include statistical analysis, machine learning, and other specialized areas. These topics involve advanced algorithms, modeling techniques, and methods for analyzing and interpreting complex data.